Table of Contents
Everything is an object
In our life, we are always surrounded by objects: you drive a car, watch TV, read books, pay for bananas with your smartphone. In Kotlin, every time when you work with variables, you work with objects. For example, an integer 5 and a string “love” are objects. It is convenient because programmers are people, and people are used to dealing with objects. In this topic, we will learn about the inner structure of objects, their state and behavior, and their distinctive features.
State and behavior
Objects are complex structures that can store some data and do something. How to get an object? Well, an object is a part of memory that stores some sort of information. Variables and values just point to objects. So, you can work with objects with the help of variables. A simple example of an object is a String
that stores a message. Let’s take a closer look at it.
First of all, a message has a state: it contains not only a sequence of symbols but also the size of the sequence, that is, the length of the message. In Kotlin, something that allows you to access the state of an object is called a property. Just put a dot and write the name of the property after the object, and you will get what you want! Suppose you have a simple String
variable msg
: val msg = "Hi"
. There is a .length
property in a string, so msg.length
gives us 2
.
In Kotlin, some functions are bound to a specific type. This makes using the objects more logical because functions represent the behavior of those objects. These functions are also called member functions or methods. The syntax is similar: just put a dot. For example, we can repeat our message using the member function repeat()
: msg.repeat(3)
gives us "HiHiHi"
.
As you can see, even the standard String
type has a really complex inner structure.
Copying by reference
In programming, copying something is a very common operation. We use =
in almost all the cases. Let’s take a look at how it works in Kotlin using a simple analogy with a bank account.
You may have several cards connected to one account. If you buy something with one of these cards, you still spend money from that one bank account, even though the cards are different.
In Kotlin, similar rules apply. If you create a variable and assign an object to it, another variable can point to the same object as well. Say you wrote text messages like this:
val msg1 = "Hi"
val msg2 = msg1
There will be two values pointing to a single object “Hi”:
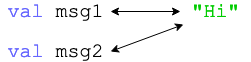
In other words, the =
sign does not copy the object itself, it only copies a reference to it.
Mutability
What happens if you change an object assigned to multiple variables by changing the value of one of these variables? It depends on the type of object. An object can be either mutable or immutable.
If the object is immutable, you cannot change it, but you can use another object and assign this new object to the same variable. When you reassign the variable, it will point to a new object and other variables will still point to the old object. Standard types such as strings or numbers are immutable, so it’s safe to copy them by reference.
The behavior of mutable objects is similar to a bank account and cards. If you modify an object from one variable, the other assigned variables continue to point to that object, so they do not save the previous data. If you buy something, you are spending money from your account and the changes will be reflected on all other cards linked to the same account.
Conclusion
Let’s recap the main points of the topic:
- Objects are complicated structures with states, properties, and member functions.
- An object is a part of memory that stores something.
- Variables only point to objects.
- Objects can be mutable or immutable.
- The only way to change something in a variable bound to an immutable object is reassigning it.
- You can freely copy immutable objects such as standard types.
- Be very careful trying to copy a mutable object.