For filtering by list and using IN operator in django-filters, you can do implement custom filter to do that.
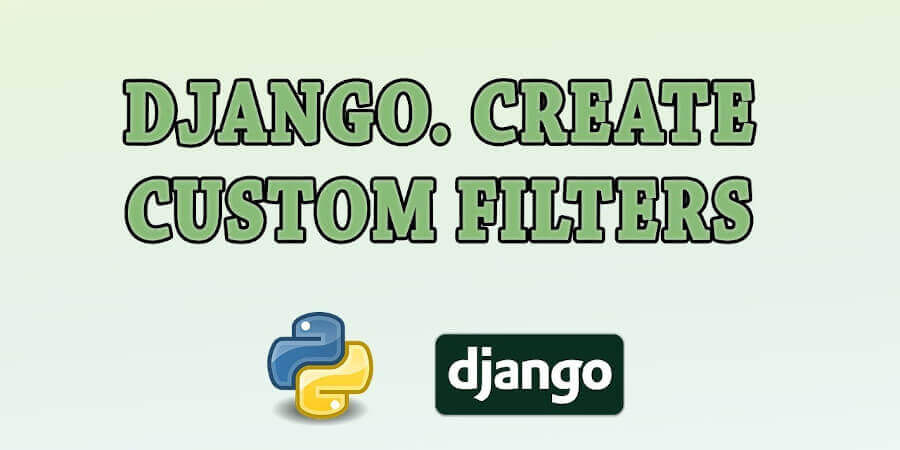
For filtering by list and using IN operator in django-filters, you can do implement custom filter to do that.
How to compare algorithms? Suppose, there is a number of algorithms solving a problem. How to compare which one is faster? One of the solutions is to run these algorithms multiple times and compare the average time used for each of the runs. However, algorithms can be written in different programming languages or they can run on computers with different architectures. So results may be different.…
In the previous topic, we started discussing pseudocode and covered some basics concepts, such as variables, arithmetic operations, conditional statements, and some others. However, some algorithms require more complex constructions to be described. In this topic, we will learn more advanced concepts of our pseudocode, such as loops, arrays and functions. Being familiar with them will allow you to express more sophisticated algorithmic ideas in…
Divide and conquer is an algorithm design paradigm in which a problem is divided into smaller subproblems (often two ones) of the same type and then each subproblem is solved independently. The division is applied recursively until sub-problems become simple enough to be solved directly using a base case. Finally, the solutions of all sub-problems are combined to get the solution for the original problem. Let’s…
In short, recursion in programmingis when a function calls itself. It has a case where it terminates and a set of rules to reduce other cases to the first case. A function that can do it is called a recursive function. Sounds a little abstract? Let’s try to get the main idea on the example. Recursive matryoshka Think of it as a Russian doll, matryoshka. It’s a doll, or,…
Let’s face it: there’s no way around algorithms if you’re hoping to write an innovative and efficient program. However, algorithms are not the only thing you need: besides the question of processing, there’s also the question of data storage. It’s best if it doesn’t take an awful lot of space, too. This is where data structures come in handy, so let’s learn the important basics…
The complexity of an algorithm Suppose you’d like to apply one of several algorithms to solve a problem. How to figure out which algorithm is better? You might implement these algorithms, start many times, and then analyze the time of solving the problem. However, this approach compares implementations rather than algorithms. There is no guarantee that the results will be the same on another computer.…
Everyday algorithms You have probably heard something about algorithms in real life. Simply, it is just a step-by-step sequence of actions that you need to do to achieve a useful result. It can be an algorithm for cooking a sandwich described by a recipe, or an algorithm for getting dressed according to today’s weather and your mood.