In this topic, you will learn how we can read information from the user input and interact with it. Quite often, programs prompt the user for some specific information. We will also see how to manage variables with the function readln() and work with different types.
Boolean and logical operations
Boolean type The boolean is a data type that has only two possible values: false and true. This is also known as the logical type.
Characters
The char type is used to represent letters (both uppercase and lowercase), digits, and other symbols. Each character is just a symbol enclosed in single quotes.
Bitwise and bit-shift operations
As you probably know, in most cases, computer numbers are represented in binary format. In this format, each digit of a number can be represented as either 0 or 1. For example, the decimal value 15 is 1111 in binary format. We’ve already learned how to convert integer numbers to binary and perform some arithmetic operations with binary numbers. Now let’s learn how else you can work with binary numbers!
Switch statement
You already know how to shape the control flow of a program using if-else statements. Perhaps, you have faced situations when you had to stack and nest multiple if-else statements to get the desired result. In this topic, you will learn an alternative way to deal with multiple choices.
Switch expression
Java 12 introduced a new feature into the Java language called switch expressions, which can be used to simplify many switch statements. Switch statements are often used to avoid long chains of if and else if statements, generally making your code more readable.
Multiple constructors
Sometimes we need to initialize all fields of an object when creating it, but there are cases in which it might be appropriate to initialize only one or several fields. Fortunately, for this purpose, a class can have several constructors that assign values to the fields in different ways. In this topic, you will learn how to work with multiple constructors and define the way…
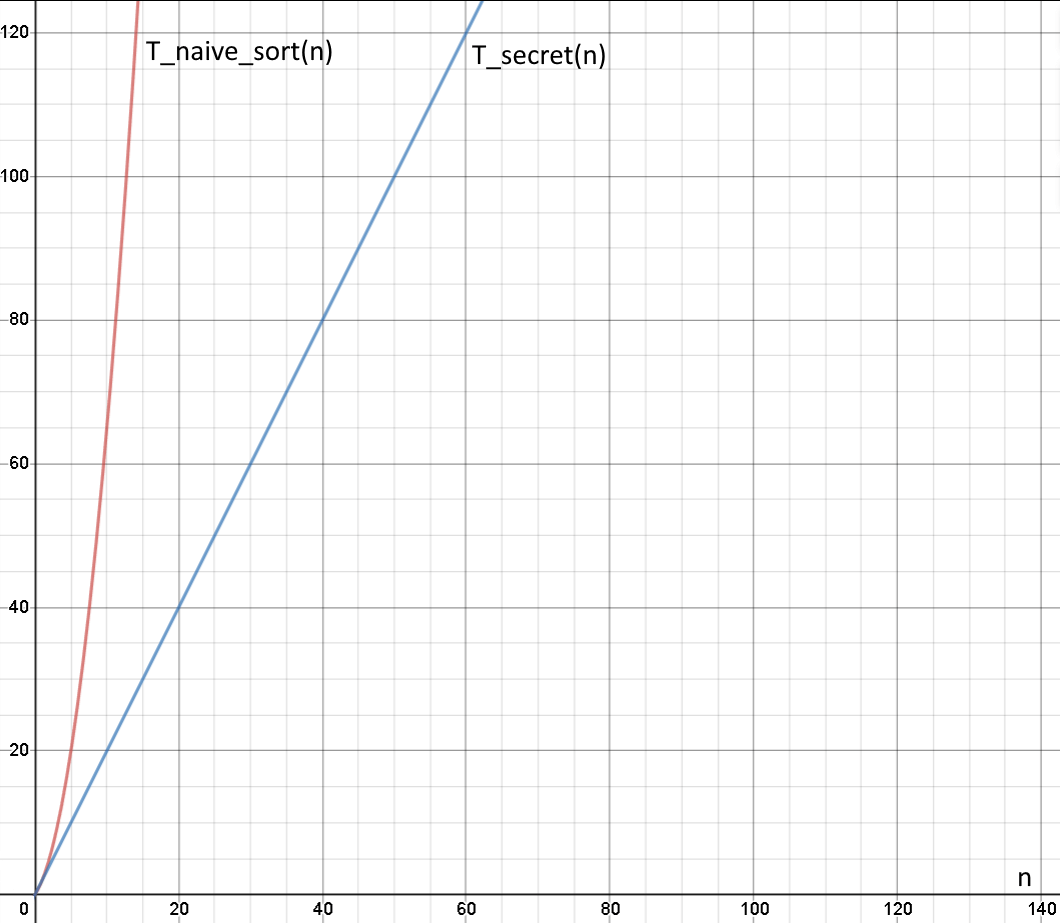
Time complexity function
How to compare algorithms? Suppose, there is a number of algorithms solving a problem. How to compare which one is faster? One of the solutions is to run these algorithms multiple times and compare the average time used for each of the runs. However, algorithms can be written in different programming languages or they can run on computers with different architectures. So results may be different.…
Complex constructions in pseudocode
In the previous topic, we started discussing pseudocode and covered some basics concepts, such as variables, arithmetic operations, conditional statements, and some others. However, some algorithms require more complex constructions to be described. In this topic, we will learn more advanced concepts of our pseudocode, such as loops, arrays and functions. Being familiar with them will allow you to express more sophisticated algorithmic ideas in…
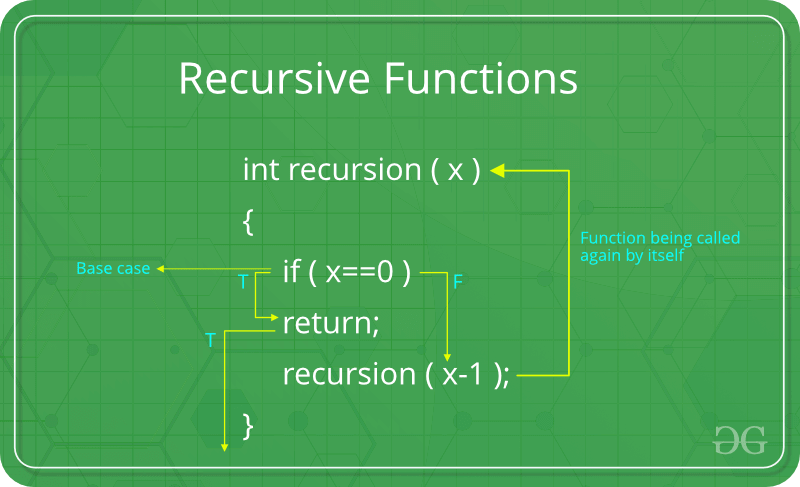
Recursion basics
In short, recursion in programmingis when a function calls itself. It has a case where it terminates and a set of rules to reduce other cases to the first case. A function that can do it is called a recursive function. Sounds a little abstract? Let’s try to get the main idea on the example. Recursive matryoshka Think of it as a Russian doll, matryoshka. It’s a doll, or,…